前端框架vue.js系列教程(7)-vue.js使用Fetch API访问RESTful API接口示例
2023-04-13 08:47:11.0
前端框架vue.js系列教程:
- 安装配置node.js和npm
- vue.js工程项目创建
- vue.js框架应用开发
- vue.js单页面应用开发
- vue.js中实现echarts绘图
- vue.js远程访问RESTful API接口示例
- vue.js使用Fetch API访问RESTful API接口示例
在本教程中,将学习在Vue 3应用程序中使用JavaScript的Fetch API来获取数据和使用REST API。
通常情况下,用户需要在前端应用程序中获取数据或消费REST API。Vue3中有内置和外部的库来发送HTTP请求到服务器,比如Fetch API和Axios HTTP客户端。在前面的教程中,我们已经掌握如何使用Axios http客户端进行Ajax请求以消费RESTful API。在本教程中,我们将step by step地学习如何在Vue 3应用程序中使用Fetch API。
什么是Fetch API?
Fetch API提供了一个JavaScript接口,用于访问和操作HTTP管道的部分,比如请求和响应。它还提供了一个全局fetch()方法,该方法提供了一种通过网络异步获取资源的简单、合理的方法。
简单来说,Fetch API是XMLHttpRequest的现代化替代品,它提供了更强大、更灵活的特性集。使用Fetch API可以快速呈现来自服务器的数据,它支持跨源资源共享(CORS)。
在Vie 3中使用Fetch API请求RESTful API接口
请按以下步骤操作。(继续使用之前创建的hellovue-app项目)
(1) 创建一个Vue页面文件。
在项目的src/views/文件夹下,创建一个名为Fetch.vue的文件,并编辑内容如下:
<template> <h2>Vue.js请求RESTful API并显示返回的数据</h2> <div class="right"> <!-- 在这里显示数据 --> <p><pre>{{ items }}</pre></p> <p v-if="loading"> 加载中... </p> </template> <script> // 请求的RESTful API 接口 var url = "http://PBCP地址IP:9527/jingsai/bar/2016" export default { name: 'FetchPage', data () { return { items: '', loading: true, } }, methods:{ // 定义一个方法 fetchData: function(url, method){ // fetch请求 fetch(url, {method: method, headers: {'content-type': 'application/json'}}) .then((response) => { // 取出响应中的json数据 * return response.json(); }) .then((data) => { // 设置响应数据 this.items = JSON.stringify(data,null,"\t\t\t"); // 控制台输出请求回来的json数据 console.log(data) }) .catch(error => { // 请求失败处理 console.log(error); }) .then(() => { this.loading = false; }); } }, mounted () { // 当这个页面挂载到DOM上时执行的方法 this.loading = true; this.fetchData(url, "get") // 调用方法 } } </script> <!-- 添加"scoped"属性来限制CSS仅作用于这个组件 --> <style scoped> .left{ width:50%; } .right{ width: 100%; float:right; } </style>
注*:Response对象不直接包含实际的JSON响应体,而是整个HTTP响应的表示。因此,要从Response对象中提取JSON正文内容,需要使用json()方法,该方法返回第二个promise,该promise将解析响应正文文本为JSON的结果。
(2) 修改主组件App.vue,添加导航链接和渲染组件,代码如下:
<template> <div class="navigate"> <ul> <!--使用 router-link 组件进行导航 --> <li><router-link to="/">首页</router-link></li> <li><router-link to="/ajax">请求RESTful API接口</router-link></li> </ul> </div> <div class="pages"> <!-- 渲染在这里 --> <router-view></router-view> </div> </template> <script> export default { name: 'App', } </script> <style> #app { font-family: Avenir, Helvetica, Arial, sans-serif; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; text-align: center; color: #2c3e50; margin-top: 60px; } .navigate{ width:200px; float:left; background-color: aliceblue; } </style>
(3) 修改入口文件main.js,导入路由组件文件并指定路由映射,主要修改部分如下:
import { createApp } from 'vue' import App from './App.vue' // 导入 vue router import { createRouter, createWebHashHistory } from 'vue-router' // 1. 定义路由组件. import FetchPage from "./views/Fetch.vue" import HomePage from "./views/Home.vue" // 2. 定义一些路由映射 const routes = [ { path: '/', component: HomePage }, { path: '/fetch', component: FetchPage }, ] // 3. 创建路由实例并传递 `routes` 配置 const router = createRouter({ // 4. 内部提供了 history 模式的实现。为了简单起见,我们在这里使用 hash 模式。 history: createWebHashHistory(), routes: routes, // `routes: routes` 的缩写 }) // 5. 创建并挂载根实例 // createApp(App).mount('#app') const app = createApp(App) // 确保 _use_ 路由实例使整个应用支持路由。 app.use(router) // 把App挂载到#app节点上 app.mount('#app')
(4) 执行Vue应用程序。
在VS Code编辑器下方的“终端”窗口中,执行如下命令,启动开发服务器:
$ npm run serve
打开浏览器,访问http://localhost:8080/ajax,可以看到如下的界面:
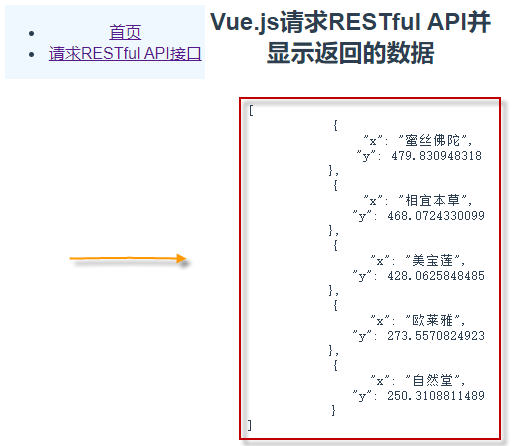
这说明我们的Vue程序已经通过Fetch API正确地请求了RESTful API接口,并接收到了返回的JSON数据。