前端框架vue.js系列教程(4)-vue.js单页面应用开发
2023-04-13 08:45:35.0
前端框架vue.js系列教程:
- 安装配置node.js和npm
- vue.js工程项目创建
- vue.js框架应用开发
- vue.js单页面应用开发
- vue.js中实现echarts绘图
- vue.js远程访问RESTful API接口示例
- vue.js使用Fetch API访问RESTful API接口示例
什么是单页面应用程序?
单页面应用(SPA,Single Page Application),就是只有一张Web页面的应用,是加载单个HTML页面并在用户与应用程序交互时动态更新该页面的web应用程序。
简单来说就是:整个Web程序只有单个HTML文件,所有操作都在这张页面上完成,由 js 来按需加载子页面内容到这张页面中,不会发生网页间的跳转。
单页面应用程序的业务逻辑全部都在本地操作,数据都需要通过 ajax 同步提交。在URL链接中采用 # 号来作为当前视图的地址(例如,http://www.xueai8.com/#),通过改变 # 号后的地址参数来载入不同的页面片段。
vue.js通过路由实现单页面程序
前端路由的概念来源于服务端,在服务端中路由描述的是 URL 与处理函数之间的映射关系。
在 Web 前端单页应用 SPA(Single Page Application)中,路由描述的是 URL 与 UI 之间的映射关系,这种映射是单向的,即 URL 变化引起 UI 更新(无需刷新页面)。
Vue 路由允许我们通过不同的 URL 访问不同的内容。通过Vue.js + vue-router(Vue的路由模块)可以很简单的实现多视图的单页Web 应用。
如果还没有安装vue-router,那么首先需要使用npm包管理器安装它,命令如下:
# 首先进入到项目根目录 $ cd E:\vueprojects\hellovue-app # 然后执行下面的安装命令 $ npm install vue-router
执行完毕,可以在项目根目录,继续执行下面的命令查看,可以看到安装的vue-router依赖包:
$ npm list
实现一个简单的vue.js单页面程序
接下来,我们继续在上一节的hellovue-app项目中实现一个简单的vue.js单页面Web应用程序。
在VS Code中打开项目文件夹,然后按以下步骤操作。
(1) 在项目的src目录下,创建一个子目录views:
(2) 在项目的src/views/目录下,分别创建三个.vue文件,分别进行编辑:
PageOne.vue:
<template> <div class="page"> <h1>我是Page One!</h1> </div> </template> <script> export default { name: 'PageOne', } </script> <style scoped> .page{ width:500px; height: 300px; background-color: brown; margin: 0 auto; line-height: 300px; color:white; } </style>
PageTwo.vue:
<template> <div class="page"> <h1>我是Page Two!</h1> </div> </template> <script> export default { name: 'PageTwo', } </script> <style scoped> .page{ width:500px; height: 300px; background-color: rgb(77, 78, 129); margin: 0 auto; line-height: 300px; color:white; } </style>
PageThree.vue:
<template> <div class="page"> <h1>我是Page Three!</h1> </div> </template> <script> export default { name: 'PageThree', } </script> <style scoped> .page{ width:500px; height: 300px; background-color: rgb(11, 82, 26); margin: 0 auto; line-height: 300px; color:white; } </style>
(3) 修改App.vue如下:
<template> <div class="navigate"> <ul> <li><router-link to="/">首页</router-link></li> <li><router-link to="/page/one">to page one</router-link></li> <li><router-link to="/page/two">to page two</router-link><</li> <li><router-link to="/page/three">to page three</router-link></li> </ul> </div> <div class="pages"> <img alt="Vue logo" src="./assets/logo.png"> <router-view></router-view> </div> </template> <script> export default { name: 'App', } </script> <style> #app { font-family: Avenir, Helvetica, Arial, sans-serif; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; text-align: center; color: #2c3e50; margin-top: 60px; } .navigate{ width:200px; float:left; background-color: aliceblue; } .pages{ margin-left: 210px; } </style>以下实例中我们将 vue-router 加进来,然后配置组件和路由映射,再告诉 vue-router 在哪里渲染它们。 注意,在上面的代码中,我们使用了两个路由相关的标签组件:
- <router-link>:该组件用于设置一个导航链接,通过传递 to属性来指定目标地址链接,即要显示的内容。router-link被解析后将呈现一个带有正确href属性的<a>标签。
- <router-view>:是路由出口,将显示与 url 对应的组件。也就是说,与roter-link标签中to属性值匹配到的组件将渲染在这里。
(4) 修改入口程序main.js,如下:
import { createApp } from 'vue' import App from './App.vue' // 导入 vue router import { createRouter, createWebHashHistory } from 'vue-router' // 1. 定义路由组件(组件名称与组件路径映射) import PageOne from "./views/PageOne.vue" import PageTwo from "./views/PageTwo.vue" import PageThree from "./views/PageThree.vue" // 2. 定义一些路由映射(路由路径与组件名称映射) const routes = [ { path: '/', component: PageOne }, { path: '/page/one', component: PageOne }, { path: '/page/two', component: PageTwo }, { path: '/page/three', component: PageThree }, ] // 3. 创建路由实例并传递 `routes` 配置 const router = createRouter({ // 4. 内部提供了 history 模式的实现 history: createWebHashHistory(), routes: routes, // 指定使用的路由 }) // 5. 创建并挂载根实例 // createApp(App).mount('#app') // 不用这个 const app = createApp(App) // 确保 _use_ 路由实例使整个应用支持路由。 app.use(router) // 把App挂载到#app节点上 app.mount('#app')
(5) 至此,我们的代码实现已经完成,接下来启动服务器。在VS Code编辑器下方的“终端”窗口中,执行如下命令:
$ npm run serve
然后打开浏览器,访问http://localhost:8080,可以看到如下的界面,这是默认首页,我们配置它和/page/one链接都指向PageOne.vue组件。
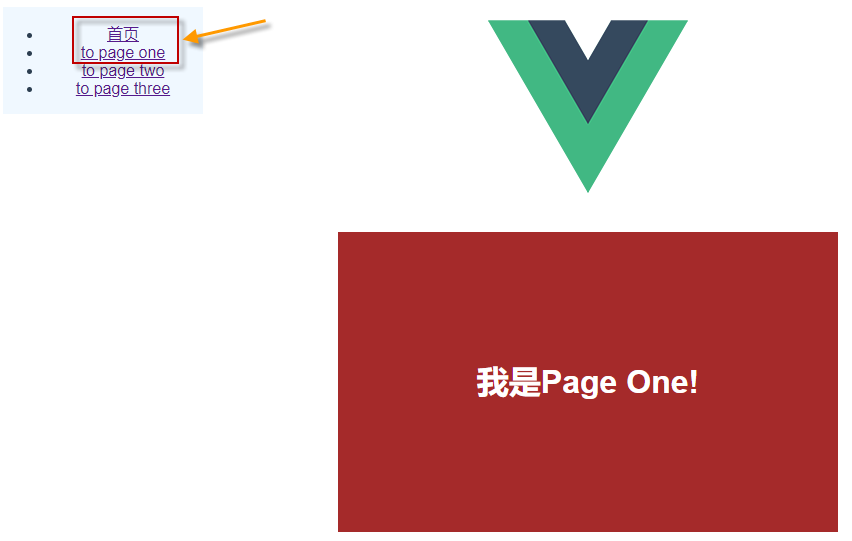
单击"to page two"链接,页面显示如下:
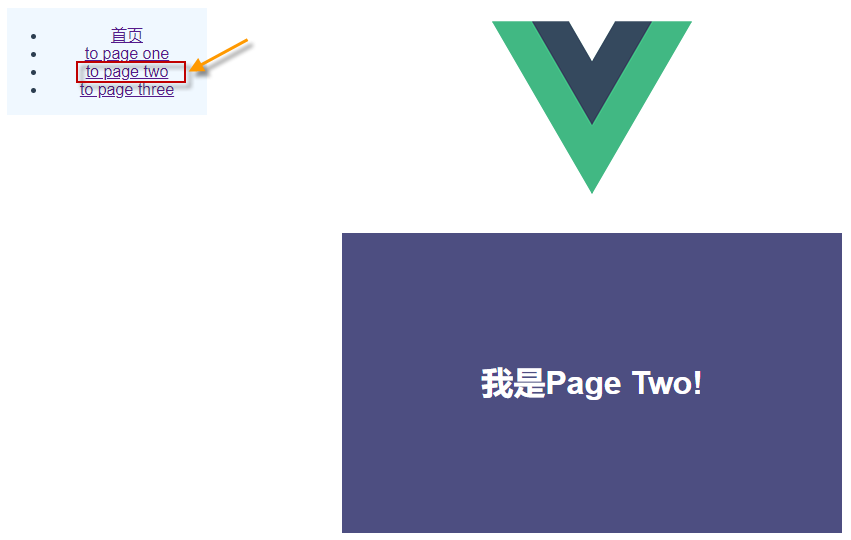
单击"to page three"链接,页面显示如下:
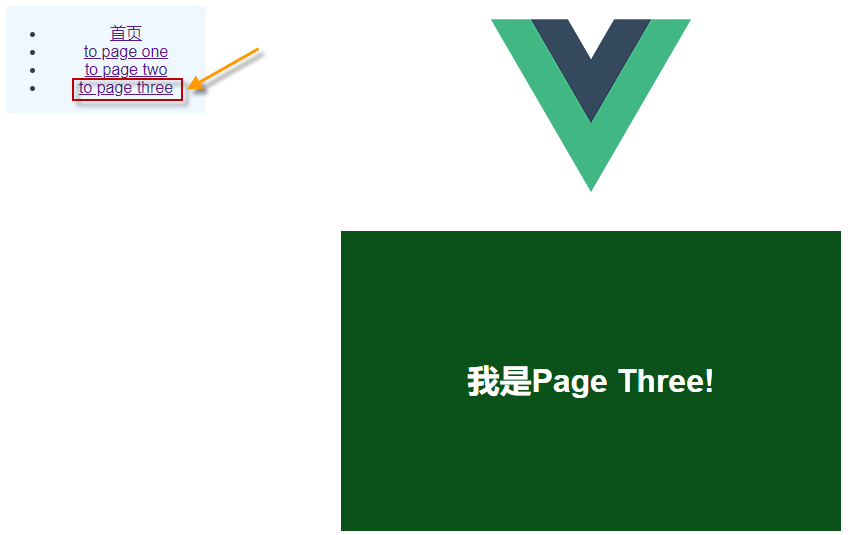
可以发现,在单击不同的导航链接切换时,并没有发生页面的跳转,一切都在是单个页面中进行的-这就是单页面应用!